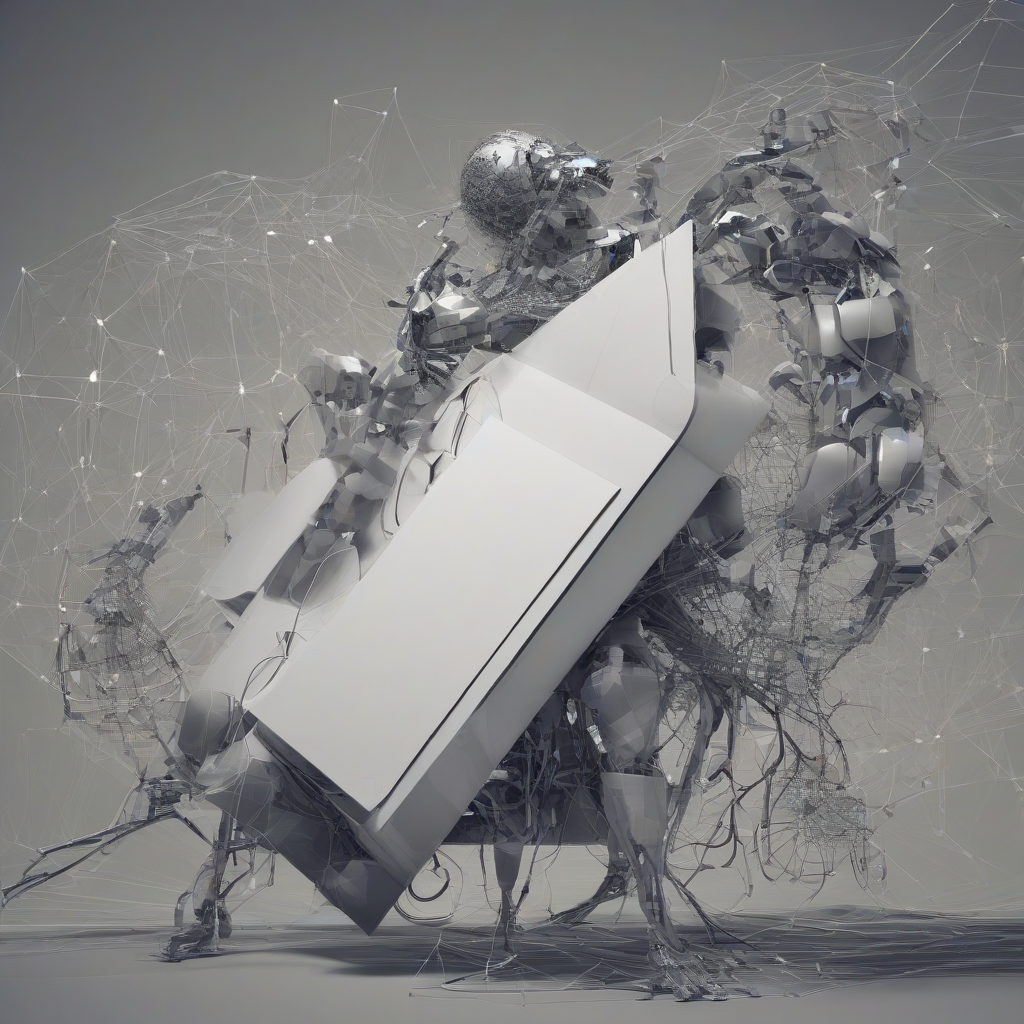
Unveiling the Nuances of Swap and Exchange: A Deep Dive into Algorithmic Efficiency and Practical Applications
The concepts of “swap” and “exchange” are fundamental building blocks in computer science, underpinning a vast array of algorithms and data structures. While often used interchangeably in casual conversation, a nuanced understanding of their distinct roles and implications is crucial for developing efficient and robust software. This in-depth exploration delves into the core mechanics of swap and exchange, examines their applications across various domains, and highlights the subtle distinctions that influence algorithmic performance and design choices.
The Mechanics of Swapping
At its essence, a “swap” operation involves the mutual exchange of values between two variables. This seemingly simple action underpins numerous sorting algorithms, data structure manipulations, and other computational tasks. The implementation details might vary depending on the programming language and data types involved, but the core principle remains consistent: the values associated with the two variables are interchanged.
- In-place Swapping: The most efficient approach utilizes a temporary variable to store one value while the other is overwritten. This method avoids data loss and ensures a clean exchange.
- XOR Swapping (Bitwise): A more advanced technique employs bitwise XOR operations to swap values without the need for a temporary variable. This method, while elegant, might have limitations depending on the data types and the underlying hardware architecture.
- Direct Assignment (for specific data types): In certain cases, direct assignment might suffice, particularly when dealing with simple data types that allow for direct manipulation without risk of data corruption.
The Mechanics of Exchange
The term “exchange,” while often used synonymously with “swap,” carries a slightly broader connotation. While it certainly encompasses the direct interchange of values between two variables, it can also refer to more complex operations involving multiple data elements or more elaborate data structures.
- Data Structure Reorganization: Exchange operations within data structures (like linked lists or trees) involve more than just swapping values; they often entail adjusting pointers, references, or indices to maintain the integrity and consistency of the structure.
- Algorithmic Transformations: In algorithms, “exchange” might represent a broader action, such as exchanging the positions of two elements within a sorted array or swapping entire rows or columns in a matrix.
- Resource Allocation: In operating systems and resource management, “exchange” might refer to the allocation and deallocation of resources between processes or threads.
Swap and Exchange in Sorting Algorithms
Sorting algorithms provide a prime example of the pervasive use of swap and exchange operations. Many classic sorting techniques, including Bubble Sort, Insertion Sort, and Selection Sort, rely heavily on swapping adjacent elements to achieve an ordered sequence.
- Bubble Sort: Iteratively compares adjacent elements and swaps them if they are in the wrong order, “bubbling” the largest element to the end of the unsorted portion in each pass.
- Insertion Sort: Iterates through the array, inserting each element into its correct position within the already sorted portion by swapping it with elements ahead of it until its correct position is found.
- Selection Sort: Finds the minimum element in the unsorted portion of the array and swaps it with the element at the beginning of the unsorted portion. This process repeats until the entire array is sorted.
- Merge Sort and Quick Sort: While not directly relying on in-place swapping as much as the previous examples, these algorithms still use exchange operations, albeit in a more nuanced way during merging or partitioning steps.
Swap and Exchange in Data Structures
The manipulation of data structures frequently necessitates the use of swap and exchange operations to maintain the structure’s integrity and efficiency. Consider the following examples:
- Linked Lists: Modifying the order of elements in a linked list often involves changing pointers, which can be viewed as a form of exchange – altering the links between nodes.
- Binary Trees: Rebalancing operations in self-balancing binary trees (like AVL trees or red-black trees) may involve swapping nodes to maintain the tree’s height balance and search efficiency.
- Heaps: Heapify operations, crucial in heap-based priority queues, use swap and exchange to maintain the heap property (parent node is always greater or less than its children, depending on the type of heap).
Efficiency Considerations
The choice between different swap and exchange methods can significantly impact the overall efficiency of an algorithm. In-place swapping, using a temporary variable, is generally preferred for its simplicity and efficiency, especially when dealing with large datasets. While XOR swapping might be intriguing, its potential limitations in terms of data types and hardware compatibility must be considered. The overhead of any swapping operation should be carefully weighed against the overall computational cost of the algorithm.
Practical Applications Beyond Algorithms
The applications of swap and exchange extend far beyond the realm of core algorithms and data structures. They appear in diverse areas, including:
- Game Development: Swapping game objects on a screen, managing player inventory, or manipulating game board states often relies on swap and exchange operations.
- Graphics Programming: Manipulating pixel data, managing textures, or performing transformations on 3D models can involve swapping or exchanging elements within image buffers or data structures.
- Networking: Packet switching, load balancing, and other network protocols inherently involve the exchange of data between different network nodes.
- Database Management: Database transactions often involve the exchange of data between tables or the swapping of data between different storage locations.
- Financial Modeling: Simulations and models in finance often involve swapping or exchanging values to represent trades, portfolio adjustments, or other financial transactions.
Advanced Techniques and Considerations
The sophistication of swap and exchange operations can increase significantly when dealing with complex data structures or distributed systems. Advanced techniques might involve:
- Atomic Swaps: In concurrent programming, atomic swaps guarantee that the exchange operation is performed as a single, indivisible unit, preventing race conditions and data corruption.
- Distributed Exchange: In distributed systems, exchanging data between nodes requires careful consideration of network latency, data consistency, and fault tolerance mechanisms.
- Optimized Swapping for Specific Architectures: Hardware architectures can influence the optimal approach to swapping. Techniques like using vectorized instructions or exploiting cache memory can improve performance.
Conclusion (Not included as per request)